It’s very easy and simple. We need to Create Database for storing the user data. and write PHP script to get user data from the form and stored into database. We have provided all files and DB below of this blog.
Let’s Create Database Using PhpMyadmin
- Go to in phpmyadmin (localhost/phpmyadmin)
- Login into phpmyadmin (Default user: root ,and pass: (NULL))
- After redirected on phpmyadmin dashboard will look like this.
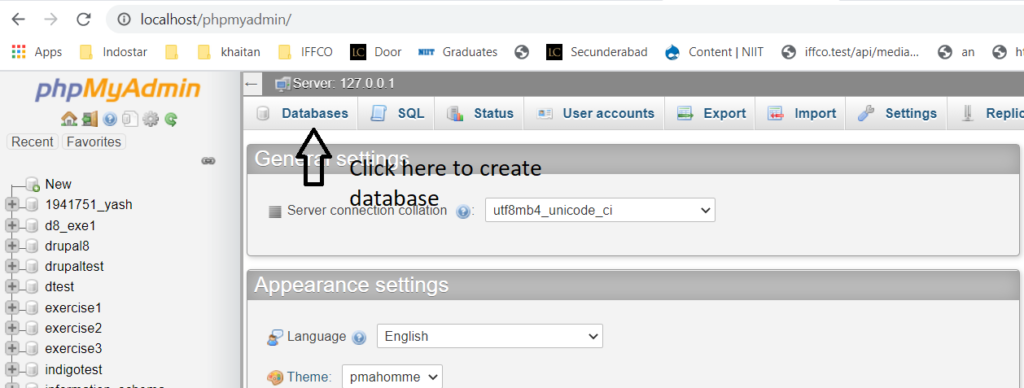
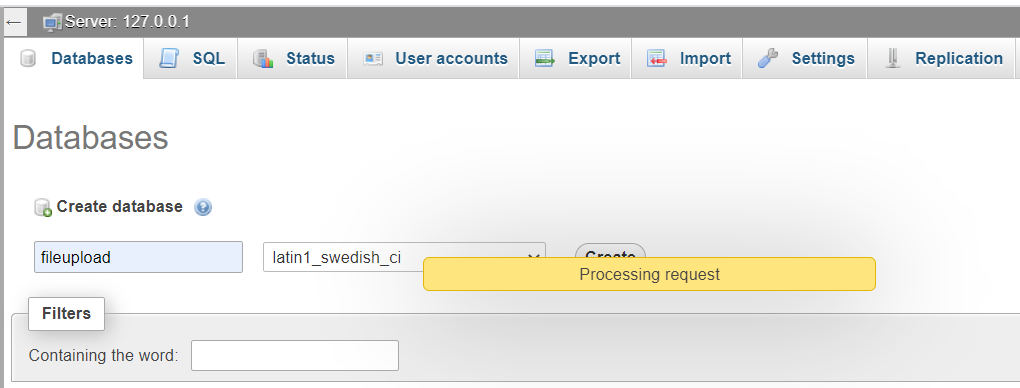
- Create table in this database here “users“
- Put numbers of field you want to create here “4″
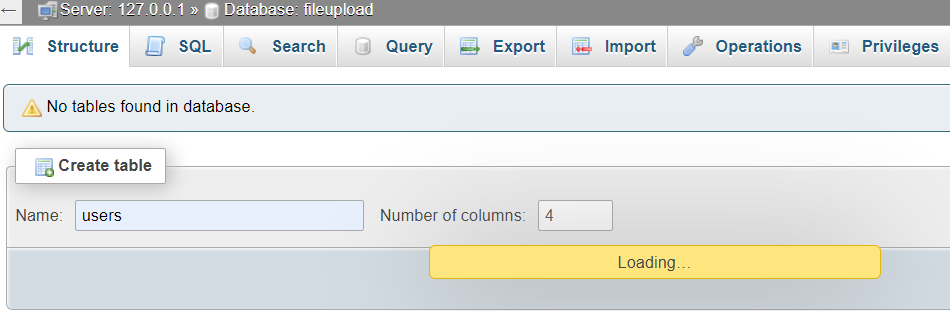
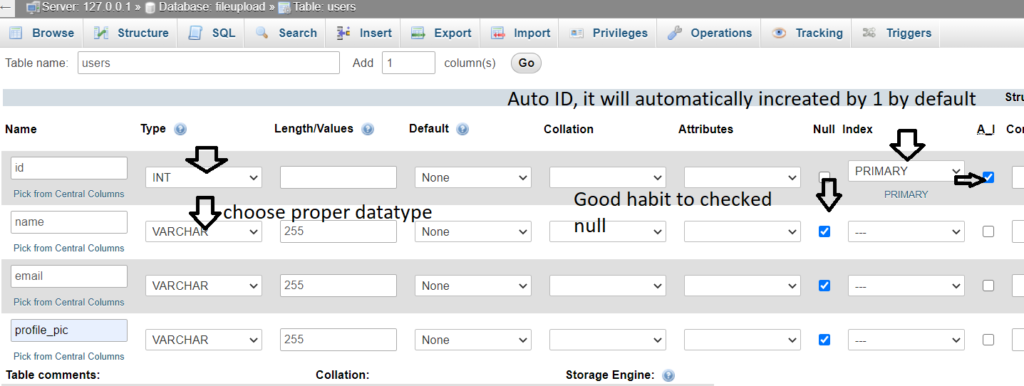
Write PHP script, to upload image and get stored into DB
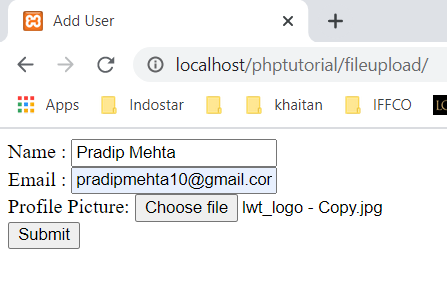
File name: index.php
<!DOCTYPE html>
<html>
<head><title>Add User</title></head>
<body>
<form action="upload.php" method="post" enctype="multipart/form-data">
Name : <input required="required" type="text" name="name" id="name"><br/>
Email : <input required="required" type="text" name="email" id="email"><br/>
Profile Picture: <input required="required" type="file" name="profile_pic" id="profile_pic"><br/>
<input type="submit" value="Submit" name="submit">
</form>
</body>
</html>
FIle Name upload.php
<?php
if(isset($_POST))
{
$name = $_POST['name'];
$email = $_POST['email'];
$to_upload_path = "";
if(isset($_FILES) && !empty($_FILES))
{
$filename = $_FILES["profile_pic"]["name"];
$to_upload_path = "uploads/".$filename;
// uploads folder must be inside your project root directory
move_uploaded_file($_FILES["profile_pic"]["tmp_name"], $to_upload_path);
}
$servername = "localhost";
$database = "fileupload";
$username = "root";
$password = "";
// Create connection
$conn = new mysqli($servername, $username, $password,$database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// write sql query for inserting data into users table.
$sql = "INSERT INTO users (name, email, profile_pic)
VALUES ('$name','$email', '$to_upload_path')";
if ($conn->query($sql) === TRUE) {
header("Location:user_list.php?q=save");
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
}
?>
- Here, we have created a directory “uploads” inside project root
- and uploaded images inside this and stores it’s path in Data users table (image name with path in this variable : $to_upload_path), so that we can get it on list page.
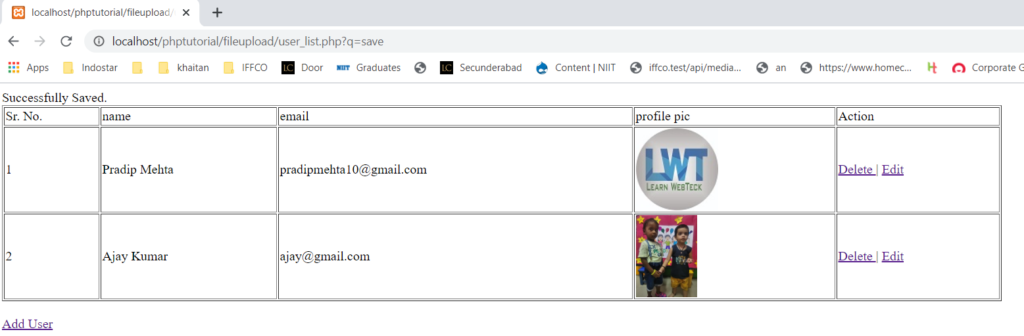
<?php
// flag to print action message
if(isset($_GET['q']) && $_GET['q']=='save')
{
echo "Successfully Saved.";
}
if(isset($_GET['q']) && $_GET['q']=='deleted')
{
echo "Successfully Deleted";
}
if(isset($_GET['q']) && $_GET['q']=='update')
{
echo "Successfully Updated";
}
// flag to print action message end
// database connection, to get user data along with images
$servername = "localhost";
$database = "fileupload";
$username = "root";
$password = "";
// Create connection
$conn = new mysqli($servername, $username, $password,$database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// write sql query for inserting data into users table.
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {?>
<table border="1" width="80%">
<tr>
<td>Sr. No.</td>
<td>name</td>
<td>email</td>
<td>profile pic</td>
<td>Action</td>
</tr>
<?php $k = 1;
while($row = $result->fetch_assoc()) { ?>
<tr>
<td><?php echo $k++;?></td>
<td><?php echo $row['name'];?></td>
<td><?php echo $row['email'];?></td>
<td><img height="100" src="<?php echo $row['profile_pic'];?>" ></td>
<td><a href="delete.php?id=<?php echo $row['id'];?>"> Delete </a> | <a href="update_profile.php?id=<?php echo $row['id'];?>"> Edit </a></td>
</tr>
<?php }
echo "<table/>";
}
?>
<br/>
<a href="index.php"> Add User</a>
- Fetch all records from Database
- for Image we get path from database e.g. uploads/Adeep.jpg and locally we have uploads directory, where all images inside this. So we are able to show images.
Let’s try to update to user data
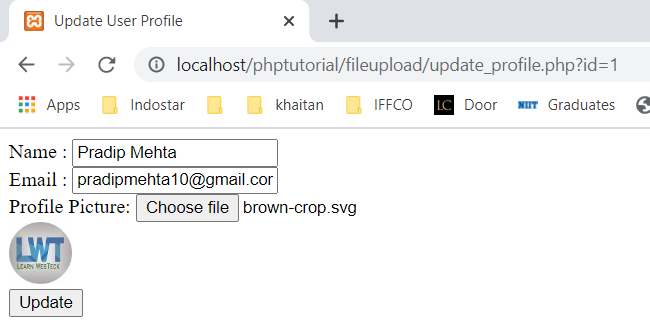
<?php
// file name update_profile.php
if(isset($_GET['id']) && $_GET['id'] > 0)
{
// database connection, to get user data along with images
$servername = "localhost";
$database = "fileupload";
$username = "root";
$password = "";
// Create connection
$conn = new mysqli($servername, $username, $password,$database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$userId = $_GET['id'];
// write sql query for inserting data into users table.
$sql = "SELECT * FROM users where id = '$userId'";
$exe_query = $conn->query($sql);
$result = $exe_query->fetch_assoc()
?>
<!DOCTYPE html>
<html>
<head><title>Update User Profile</title></head>
<body>
<form action="update.php" method="post" enctype="multipart/form-data">
Name : <input required="required" value="<?php echo $result['name'];?>" type="text" name="name" id="name"><br/>
Email : <input required="required" value="<?php echo $result['email'];?>" type="text" name="email" id="email"><br/>
Profile Picture: <input type="file" name="profile_pic" id="profile_pic"> <br/>
<img height="50" src="<?php echo $result['profile_pic'];?>">
<input type="hidden" name="profile_pic_update" value="<?php echo $result['profile_pic'];?>">
<input type="hidden" name="id" value="<?php echo $result['id'];?>">
<br/>
<input type="submit" value="Update" name="submit">
</form>
</body>
</html>
<?php }?>
File name : update.php (update_profile controller)
<?php
if(isset($_POST))
{
$name = $_POST['name'];
$email = $_POST['email'];
$id = $_POST['id'];
$to_upload_path = "";
if(isset($_FILES) && !empty($_FILES))
{
$filename = $_FILES["profile_pic"]["name"];
$to_upload_path = "uploads/".$filename;
if(move_uploaded_file($_FILES["profile_pic"]["tmp_name"], $to_upload_path))
{
}else{
$to_upload_path = $_POST['profile_pic_update'];
}
}
$servername = "localhost";
$database = "fileupload";
$username = "root";
$password = "";
// Create connection
$conn = new mysqli($servername, $username, $password,$database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// write sql query for inserting data into users table.
$sql = "update users set name = '$name', email = '$email' , profile_pic ='$to_upload_path' where id = '$id'";
if ($conn->query($sql) === TRUE) {
header("Location:user_list.php?q=update");
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
}
?>
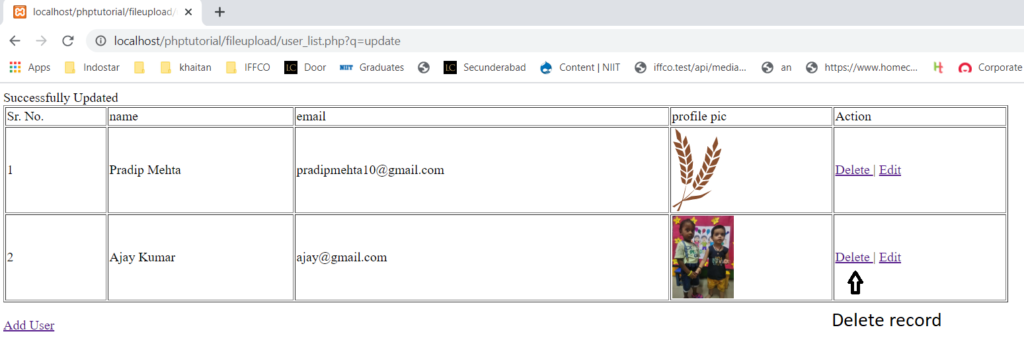
<?php
if(isset($_GET['id']))
{
$servername = "localhost";
$database = "fileupload";
$username = "root";
$password = "";
// Create connection
$conn = new mysqli($servername, $username, $password,$database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$userId = $_GET['id'];
// write sql query for inserting data into users table.
$sql = "delete from users where id = '$userId'";
if ($conn->query($sql) === TRUE) {
header("Location:user_list.php?q=deleted");
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
}
?>
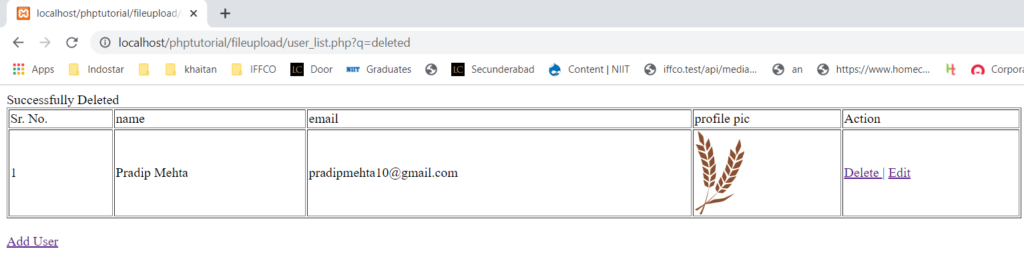
How to setup on your local system ?
- To Download the code click here
- Check carefully, DB user name and password
- Import Database in your local DB
- Your file structure will look like below.
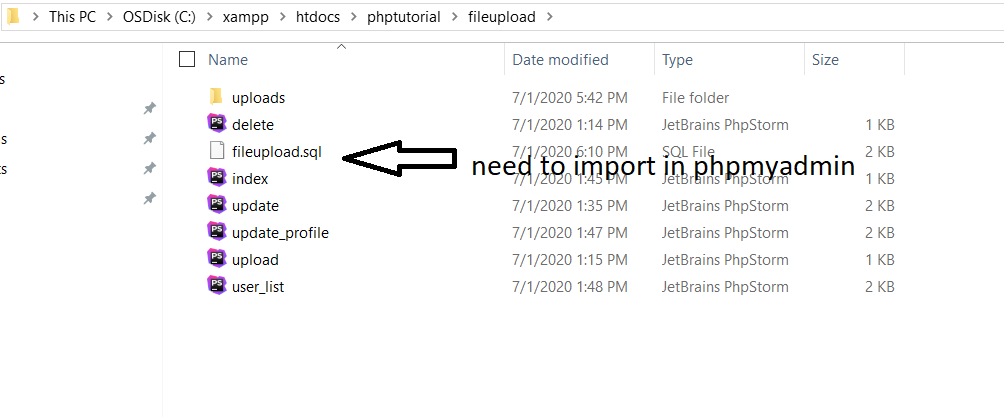
How to import sql file in Database
- Login in phpmyadmin
- create database
- Click on import
- Browse the SQL file
- Click on Go
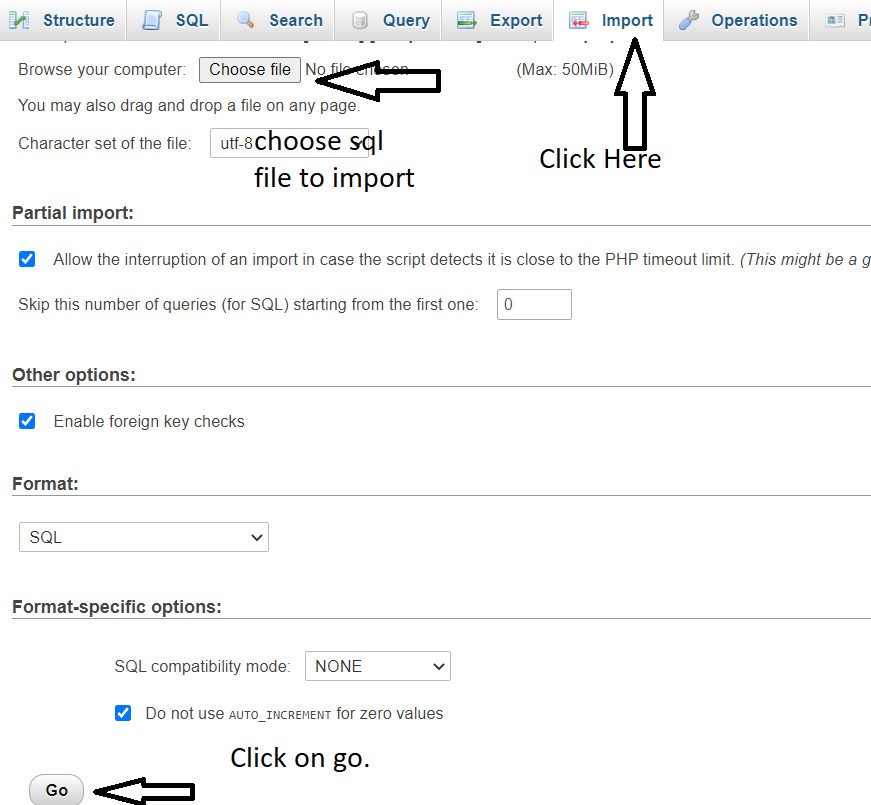